1. 概述
简而言之,JCache是Java的标准缓存API。在本教程中,我们将了解JCache是什么以及如何使用它。
2. Maven依赖
要使用JCache,我们需要在pom.xml中添加以下依赖:
<dependency>
<groupId>javax.cache</groupId>
<artifactId>cache-api</artifactId>
<version>1.1.1</version>
</dependency>
请注意,我们可以在Maven Central中找到该库的最新版本。
我们还需要在pom.xml中添加API的实现;我们将在这里使用Hazelcast:
<dependency>
<groupId>com.hazelcast</groupId>
<artifactId>hazelcast</artifactId>
<version>5.2.0</version>
</dependency>
也可以在Maven Central中找到Hazelcast的最新版本。
3. JCache实现
JCache由各种缓存解决方案实现:
- JCache参考实现
- Hazelcast
- Oracle Coherence
- Terracotta Ehcache
- Infinispan
- Redisson
- Apache Ignite
请注意,与其他参考实现不同,不建议在生产中使用JCache参考实现,因为它会导致一些并发问题。
4. 主要组件
4.1 Cache
Cache接口有以下有用的方法:
- get():以元素的键作为参数并返回元素的值;如果缓存中不存在该键,则返回null
- getAll():可以将多个键作为Set传递给此方法;该方法将给定的键和相关值作为Map返回
- getAndRemove():该方法使用其键检索值并从缓存中删除元素
- put():在缓存中插入新元素
- clear():删除缓存中的所有元素
- containsKey():检查缓存是否包含特定键
我们可以看到,这些方法的名称非常直观。有关这些方法和其他方法的更多信息,请访问Javadoc。
4.2 CacheManager
CacheManager是API中最重要的接口之一,它使我们能够建立、配置和关闭缓存。
4.3 CachingProvider
CachingProvider是一个接口,它允许我们创建和管理CacheManagers的生命周期。
4.4 Configuration
Configuration是一个使我们能够配置Caches的接口,它有一个具体实现MutableConfiguration和一个子接口CompleteConfiguration。
5. 创建缓存
让我们看看如何创建一个简单的缓存:
CachingProvider cachingProvider = Caching.getCachingProvider();
CacheManager cacheManager = cachingProvider.getCacheManager();
MutableConfiguration<String, String> config = new MutableConfiguration<>();
Cache<String, String> cache = cacheManager
.createCache("simpleCache", config);
cache.put("key1", "value1");
cache.put("key2", "value2");
cacheManager.close();
我们所做的就是:
- 创建一个CachingProvider对象,我们将使用该对象构造一个CacheManager对象
- 创建一个MutableConfiguration对象,它是Configuration接口的实现
- 使用我们之前创建的CacheManager对象创建Cache对象
- 把所有需要缓存的条目放入我们的Cache对象中
- 关闭CacheManager以释放缓存使用的资源
如果我们没有在pom.xml中提供JCache的任何实现,则会抛出以下异常:
javax.cache.CacheException: No CachingProviders have been configured
原因是JVM找不到getCacheManager()方法的任何具体实现。
6. EntryProcessor
EntryProcessor允许我们使用原子操作修改Cache条目,而不必将它们重新添加到Cache中。要使用它,我们需要实现EntryProcessor接口:
public class SimpleEntryProcessor implements EntryProcessor<String, String, String>, Serializable {
public String process(MutableEntry<String, String> entry, Object... args) throws EntryProcessorException {
if (entry.exists()) {
String current = entry.getValue();
entry.setValue(current + " - modified");
return current;
}
return null;
}
}
现在,让我们使用EntryProcessor实现:
@Test
public void whenModifyValue_thenCorrect() {
this.cache.invoke("key", new SimpleEntryProcessor());
assertEquals("value - modified", cache.get("key"));
}
7. 事件监听器
事件监听器允许我们在触发EventType枚举中定义的任何事件类型时采取行动,这些事件类型包括:
- CREATED
- UPDATED
- REMOVED
- EXPIRED
首先,我们需要实现我们将要使用的事件的接口。
例如,如果我们想使用CREATED和UPDATED事件类型,那么我们应该实现接口CacheEntryCreatedListener和CacheEntryUpdatedListener。
我们来看一个例子:
public class SimpleCacheEntryListener implements
CacheEntryCreatedListener<String, String>,
CacheEntryUpdatedListener<String, String>,
Serializable {
private boolean updated;
private boolean created;
// standard getters
public void onUpdated(Iterable<CacheEntryEvent<? extends String,
? extends String>> events) throws CacheEntryListenerException {
this.updated = true;
}
public void onCreated(Iterable<CacheEntryEvent<? extends String,
? extends String>> events) throws CacheEntryListenerException {
this.created = true;
}
}
现在,让我们运行测试:
@Test
public void whenRunEvent_thenCorrect() throws InterruptedException {
this.listenerConfiguration = new MutableCacheEntryListenerConfiguration<String, String>(
FactoryBuilder.factoryOf(this.listener), null, false, true);
this.cache.registerCacheEntryListener(this.listenerConfiguration);
assertEquals(false, this.listener.getCreated());
this.cache.put("key", "value");
assertEquals(true, this.listener.getCreated());
assertEquals(false, this.listener.getUpdated());
this.cache.put("key", "newValue");
assertEquals(true, this.listener.getUpdated());
}
8. CacheLoader
CacheLoader允许我们使用读通模式,将缓存作为主数据存储并从中读取数据。
在实际场景中,我们可以让缓存从实际存储中读取数据。
我们来看一个例子。首先,我们应该实现CacheLoader接口:
public class SimpleCacheLoader implements CacheLoader<Integer, String> {
public String load(Integer key) throws CacheLoaderException {
return "fromCache" + key;
}
public Map<Integer, String> loadAll(Iterable<? extends Integer> keys) throws CacheLoaderException {
Map<Integer, String> data = new HashMap<>();
for (int key : keys) {
data.put(key, load(key));
}
return data;
}
}
现在,让我们使用我们的CacheLoader实现:
public class CacheLoaderIntegrationTest {
private Cache<Integer, String> cache;
@Before
public void setup() {
CachingProvider cachingProvider = Caching.getCachingProvider();
CacheManager cacheManager = cachingProvider.getCacheManager();
MutableConfiguration<Integer, String> config
= new MutableConfiguration<>()
.setReadThrough(true)
.setCacheLoaderFactory(new FactoryBuilder.SingletonFactory<>(
new SimpleCacheLoader()));
this.cache = cacheManager.createCache("SimpleCache", config);
}
@Test
public void whenReadingFromStorage_thenCorrect() {
for (int i = 1; i < 4; i++) {
String value = cache.get(i);
assertEquals("fromCache" + i, value);
}
}
}
9. 总结
在本教程中,我们了解了JCache是什么,并在一些实际场景中探讨了它的一些重要功能。
Post Directory
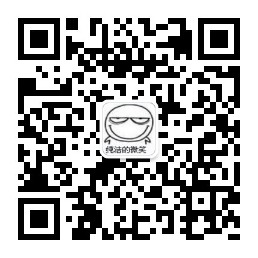