1. 简介
Derive4J是一个注解处理器,它支持Java 8中的各种函数概念。
在本教程中,我们将介绍Derive4J和该框架支持的最重要的概念:
- 代数数据类型
- 结构模式匹配
- 一流的惰性求值
2. Maven依赖
要使用Derive4J,我们需要将依赖包含到我们的项目中:
<dependency>
<groupId>org.derive4j</groupId>
<artifactId>derive4j</artifactId>
<version>1.1.0</version>
<optional>true</optional>
</dependency>
3. 代数数据类型
3.1 描述
代数数据类型(ADT)是一种复合类型-它们是其他类型或泛型的组合。
ADT通常分为两大类:
- sum
- product
许多语言(例如Haskell和Scala)默认存在代数数据类型。
3.2 Sum类型
Sum是代表逻辑或运算的数据类型。这意味着它可以是一回事,但不能是两回事。简单来说,Sum类型是一组不同的情况。名称“Sum”源于这样一个事实:不同值的总数就是情况的总数。
Enum是Java中最接近于sum类型的类型。Enum有一组可能的值,但一次只能有一个。但是,在Java中,我们不能将任何其他数据与Enum关联,这是代数数据类型相对于Enum的主要优势。
3.3 Product类型
Product是表示逻辑AND运算的数据类型,它是多个值的组合。
Java中的类可以看作是一种product类型,product类型由其字段的组合来定义。
我们可以在这篇维基百科文章中找到有关ADT的更多信息。
3.4 使用
常用的代数数据类型之一是Either,我们可以将Either视为更复杂的Optional,可用于存在缺失值的可能性或操作可能导致异常的情况。
我们需要用至少一种抽象方法来标注一个抽象类或接口,Derive4J将使用这种方法来生成我们的ADT结构。
为了在Derive4J中创建Either数据类型,我们需要创建一个接口:
@Data
interface Either<A, B> {
<X> X match(Function<A, X> left, Function<B, X> right);
}
我们的接口带有@Data注解,这将允许Derive4J为我们生成适当的代码。生成的代码包含工厂方法、惰性构造函数和各种其他方法。
默认情况下,生成的代码会获取注类的名称,但采用复数形式。但是,可以通过inClass参数进行配置。
现在,我们可以使用生成的代码来创建Either ADT并验证它是否正常工作:
public void testEitherIsCreatedFromRight() {
Either<Exception, String> either = Eithers.right("Okay");
Optional<Exception> leftOptional = Eithers.getLeft(either);
Optional<String> rightOptional = Eithers.getRight(either);
Assertions.assertThat(leftOptional).isEmpty();
Assertions.assertThat(rightOptional).hasValue("Okay");
}
我们还可以使用生成的match()方法根据Either的哪一侧存在来执行函数:
public void testEitherIsMatchedWithRight() {
Either<Exception, String> either = Eithers.right("Okay");
Function<Exception, String> leftFunction = Mockito.mock(Function.class);
Function<String, String> rightFunction = Mockito.mock(Function.class);
either.match(leftFunction, rightFunction);
Mockito.verify(rightFunction, Mockito.times(1)).apply("Okay");
Mockito.verify(leftFunction, Mockito.times(0)).apply(Mockito.any(Exception.class));
}
4. 模式匹配
使用代数数据类型实现的功能之一是模式匹配。
模式匹配是一种根据模式检查值的机制。基本上,模式匹配是一种更强大的switch语句,但不限制匹配类型或要求模式不变。有关更多信息,我们可以查看这篇关于模式匹配的维基百科文章。
要使用模式匹配,我们将创建一个类来模拟HTTP请求。用户将能够使用给定的HTTP方法之一:
- GET
- POST
- DELETE
- PUT
让我们将请求类建模为Derive4J中的ADT,从HTTPRequest接口开始:
@Data
interface HTTPRequest {
interface Cases<R>{
R GET(String path);
R POST(String path);
R PUT(String path);
R DELETE(String path);
}
<R> R match(Cases<R> method);
}
生成的类HttpRequests(注意复数形式)现在允许我们根据请求的类型执行模式匹配。
为此,我们将创建一个非常简单的HTTPServer类,它将根据请求的类型以不同的状态响应。
首先,让我们创建一个简单的HTTPResponse类,它将作为从我们的服务器到我们的客户端的响应:
public class HTTPResponse {
int statusCode;
String responseBody;
public HTTPResponse(int statusCode, String responseBody) {
this.statusCode = statusCode;
this.responseBody = responseBody;
}
}
然后我们可以创建使用模式匹配来发送正确响应的服务器:
public class HTTPServer {
public static String GET_RESPONSE_BODY = "Success!";
public static String PUT_RESPONSE_BODY = "Resource Created!";
public static String POST_RESPONSE_BODY = "Resource Updated!";
public static String DELETE_RESPONSE_BODY = "Resource Deleted!";
public HTTPResponse acceptRequest(HTTPRequest request) {
return HTTPRequests.caseOf(request)
.GET((path) -> new HTTPResponse(200, GET_RESPONSE_BODY))
.POST((path,body) -> new HTTPResponse(201, POST_RESPONSE_BODY))
.PUT((path,body) -> new HTTPResponse(200, PUT_RESPONSE_BODY))
.DELETE(path -> new HTTPResponse(200, DELETE_RESPONSE_BODY));
}
}
我们类的acceptRequest()方法对请求的类型使用模式匹配,并将根据请求的类型返回不同的响应:
@Test
public void whenRequestReachesServer_thenProperResponseIsReturned() {
HTTPServer server = new HTTPServer();
HTTPRequest postRequest = HTTPRequests.POST("http://test.com/post", "Resource");
HTTPResponse response = server.acceptRequest(postRequest);
Assert.assertEquals(201, response.getStatusCode());
Assert.assertEquals(HTTPServer.POST_RESPONSE_BODY, response.getResponseBody());
}
5. 一流的惰性求值
Derive4J允许我们引入惰性概念,这意味着我们的对象只有在我们对它们执行操作时才会被初始化。让我们将接口声明为LazyRequest并将生成的类配置为LazyRequestImpl:
@Data(value = @Derive(
inClass = "{ClassName}Impl",
make = {Make.lazyConstructor, Make.constructors}
))
public interface LazyRequest {
interface Cases<R>{
R GET(String path);
R POST(String path, String body);
R PUT(String path, String body);
R DELETE(String path);
}
<R> R match(LazyRequest.Cases<R> method);
}
现在我们可以验证生成的惰性构造函数是否正常工作:
@Test
public void whenRequestIsReferenced_thenRequestIsLazilyContructed() {
LazyRequestSupplier mockSupplier = Mockito.spy(new LazyRequestSupplier());
LazyRequest request = LazyRequestImpl.lazy(() -> mockSupplier.get());
Mockito.verify(mockSupplier, Mockito.times(0)).get();
Assert.assertEquals(LazyRequestImpl.getPath(request), "http://test.com/get");
Mockito.verify(mockSupplier, Mockito.times(1)).get();
}
class LazyRequestSupplier implements Supplier<LazyRequest> {
@Override
public LazyRequest get() {
return LazyRequestImpl.GET("http://test.com/get");
}
}
我们可以在Scala文档中找到有关一流惰性和示例的更多信息。
6. 总结
在本教程中,我们介绍了Derive4J库并使用它来实现一些功能概念,例如代数数据类型和模式匹配,这些概念通常在Java中不可用。
Post Directory
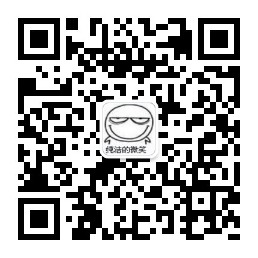